Learn Python Game Development with PyGame
In this course you'll learn how to make a substantial game from scratch. The course comes with full source code, and graphical assets.
- 10-part Course
- Full Game with Code
- Free Graphical Assets
What You Will Learn
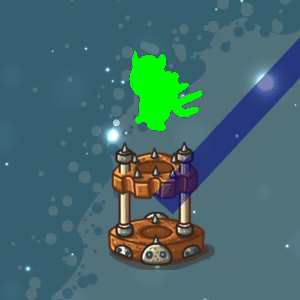
Collision Detection
Learn how to detect when two or more objects intersect. Collision detection is an essential component of nearly all games. You will learn about rectangular collision detection and bit mask collision detection — when you want to detect whether a turret should fire its arsenal, or when an enemy is struck by a projectile, you need collision detection.
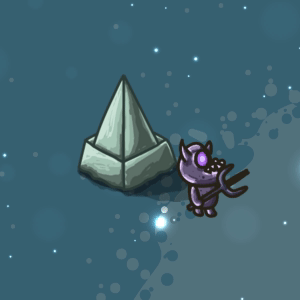
Animation
To truly bring any game to life, you need animations. You'll learn how to take a series of frames that make up an animation and render them on screen so they appear life-like.
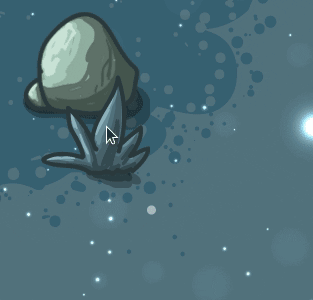
Level Editing
Build a level editor from scratch — complete with save and load functionality — with mouse-based pick and placement of graphical assets. You'll learn about tiled grid engines and also how to layer graphics so your graphical assets are rendered in the right order.
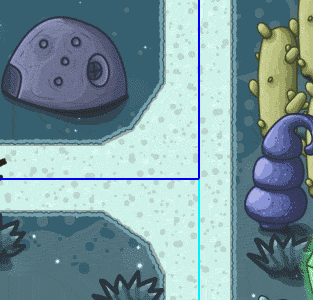
Pathfinding
You will combine graph theory and recursion to build depth-first search and implement basic path finding algorithms that can determine valid paths for the enemies in the game to walk through.
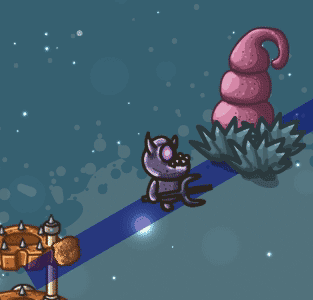
Kinematics & Vector Mathematics
Learn how to implement kinematics using linear interpolation and vector mathematics to determine how to add movement to graphics, like projectiles or moving enemies. Linear interpolation and vector mathematics form a core part of all motion-related activities you want to do in a game.
class GameState(enum.Enum):
initializing = "initializing"
initialized = "initialized"
map_editing = "map_editing"
game_playing = "game_playing"
main_menu = "main_menu"
game_ended = "game_ended"
quitting = "quitting"
Finite State Automata & State Machines
Building a maintainable game is hard; there's a lot of state, and it's easy to lose sight of which part of your code does what. That's where state machines can help. They neatly separate distinct areas of your code — what your game has to do in game play mode versus the main menu, for example — by reducing a mess of variables and complex functions into simple class structures with a single state.
def generate_turret_sweep(orientation, deg):
return cycle(
chain(
range(orientation - deg,
orientation + deg),
reversed(range(orientation - deg,
orientation + deg)),
)
)
Lazy Evaluation & Generator Expressions
Python's generators simplify complex pieces of repetitive code, such as generating paths for enemies to walk; the sweep of a turret's sights; and animations. You will learn how to use advanced Python concepts like yield
and the itertools
module to express repetitions with a few lines of code.
class Projectile(DirectedSprite):
_layer = Layer.projectile
def update(self):
super().update()
if self.state == SpriteState.stopped:
self.animation_state = AnimationState.exploding
Object-Oriented Programming
Explore advanced OOP patterns like factory class methods, Python dataclasses
and dependency injection that will greatly improve the readability and code reuse of your game code.
Build class hierarchies that you can easily extend and repurpose for other game projects.